Integrating Flutter Clean Architecture and repositories into your development workflow represents a pivotal strategy for enhancing the structure and quality of your Flutter applications.
This article aims to unravel the principles of Clean Architecture within the Flutter ecosystem, focusing specifically on the pivotal role of repositories in abstracting data sources and ensuring a decoupled, testable, and maintainable codebase.
By providing a deep dive into the repository design pattern and its application in Flutter Clean Architecture, we offer valuable insights and practical steps to developers seeking to leverage these concepts for improved app performance and scalability.
What Is the Repository Pattern?
The Repository pattern is a widely recognized design pattern in software development, serving as a bridge between the business logic of an application and its data sources.
Its primary objective is to abstract the way data is retrieved and stored, allowing the business logic to interact with a unified interface regardless of where and how the data is actually stored.
This abstraction facilitates a separation of concerns, making the code more modular, easier to test, and, ultimately, more resilient to changes in the data layer.
In the context of Clean Architecture, the Repository pattern takes on an essential role by acting as the intermediary between the application’s core logic and its data sources, such as databases or web services.
Clean Architecture, as proposed by Robert C. Martin, emphasizes the separation of concerns by organizing the code into distinct layers with clear responsibilities.
The Repository pattern fits within this architecture by residing in the interface adapters layer, ensuring that the use cases (business rules) layer remains completely isolated from the details of how data is accessed or persisted.
This isolation not only improves the maintainability and scalability of the application but also enhances its ability to adapt to changes in external data sources without significant impact on the core business logic.
Thus, the Repository pattern is not just a technical implementation detail; it’s a strategic choice that aligns with the principles of Clean Architecture to promote a more structured, maintainable, and testable codebase.
By decoupling the data access logic from the rest of the application, developers can more easily manage changes to the data storage mechanisms, optimize data access strategies, and ensure that the application’s business logic is clear and concise.
Why Are Repositories Important in Flutter’s Clean Architecture?
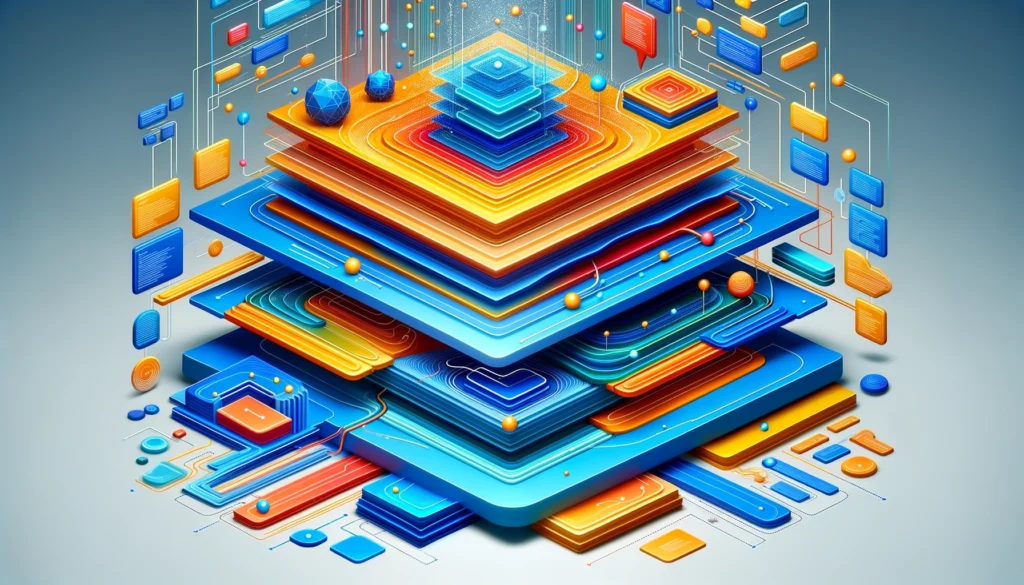
Repositories play a crucial role in implementing Clean Architecture or MVVM within Flutter applications, acting as a cornerstone for achieving a clean separation of concerns and a more maintainable, scalable codebase. Here are the key reasons why repositories are so important in Flutter’s Clean Architecture:
1. Abstraction of Data Sources
Repositories abstract away the complexity of data handling, allowing the rest of the application to interact with data through a simple, unified interface. This is particularly beneficial in Flutter, where applications often consume data from multiple sources, such as local databases, web services, or external APIs. By encapsulating the data access logic within repositories, Flutter developers can easily manage and switch between different data sources without impacting the business logic.
2. Decoupling Business Logic from Data Layer
In the Clean Architecture model, the separation of concerns is paramount. Repositories contribute to this by decoupling the business logic (use cases) from the data layer. This decoupling ensures that changes to the data source, such as migrating from one database system to another or altering the data access strategy, have minimal impact on the core business logic. For Flutter developers, this means enhanced modularity and easier maintenance.
3. Improved Testability
With repositories abstracting the data layer, testing becomes more straightforward. Developers can focus on writing unit tests for the business logic without worrying about the complexities of real data sources. In Flutter, where rapid development and frequent updates are common, repositories enable developers to mock data sources efficiently, ensuring that tests are both reliable and fast.
4. Consistency Across the Application
Repositories help maintain consistency in how data is handled across different parts of a Flutter application. By enforcing a standard approach to data access and manipulation, repositories reduce the risk of errors and inconsistencies, leading to a more robust and reliable application.
5. Facilitates Scalability
As Flutter applications grow in complexity and size, the benefits of using repositories become even more apparent. Repositories allow for scaling the application both vertically (adding more features) and horizontally (handling more data or users) by managing data interactions in a centralized, standardized way. This scalability is crucial for enterprise-level applications or those expecting to grow over time.
6. Supports Advanced Data Management Techniques
Finally, repositories in Flutter’s Clean Architecture support advanced data management techniques, such as caching, offline storage, and data synchronization. By centralizing the logic for these operations within repositories, developers can implement sophisticated data handling strategies that improve the user experience, such as reducing load times and ensuring the application remains functional even without an internet connection.
Implementing Repositories in Flutter: A Step-by-Step Guide
Project Structure and Dependencies
- Create a New Flutter Project: Start by creating a new Flutter project in your development environment. You can do this using the Flutter command line tool by running flutter create your_project_name.
- Organize Your Project into Layers: Clean Architecture involves dividing your project into layers, each with its defined responsibilities. A common structure includes:
- Handles raw data (from network requests, databases, etc.). This is where the repository implementations will reside.
- Contains business logic and use cases. It’s independent of the framework and the UI.
- Comprises UI components and state management, connecting the UI with the use cases from the domain layer.
- Add Dependencies: Your project will likely need additional packages, such as http for network requests, provider or bloc for state management, and possibly a local database package like sqflite or hive. Add these to your pubspec.yaml file.
Designing the Repository Layer in Flutter
Defining Interfaces for Repositoriesy
- Identify Data Operations: Determine what data operations your application needs to perform, such as fetching data from the network, reading from or writing to a local database, or caching data.
- Define Repository Interfaces: Create abstract classes in Dart that define the interface for these operations. For example, if you’re building a task management app, you might have a TaskRepository interface with methods like getAllTasks(), getTaskById(int id), and saveTask(Task task).
- Use Dependency Injection: To maintain the flexibility and testability of your code, use dependency injection to provide concrete implementations of these repositories to your business logic (use cases) and UI layers.
Creating Concrete Implementations of Repositories
- Implement the Interfaces: For each repository interface, create a concrete class that implements the defined methods. The implementation will handle the specifics of data access, whether it’s calling a web API or querying a local database.
- Handle Data Sources: Inside your concrete repository implementations, manage the different data sources your application uses. For instance, a TaskRepositoryImpl might have a RemoteDataSource for fetching tasks from a server and a LocalDataSource for caching tasks locally.
- Integrate with the Rest of the Application: With your repositories implemented, you can now inject them into your use cases. This allows your application’s business logic to interact with data through the abstract repository interfaces, keeping the code clean and adherent to the principles of Clean Architecture.
- Testing: Once your repositories are set up, write unit tests to verify their behavior. Mock the data sources to test how your repositories handle different scenarios, such as network failures or empty caches.
When and How to Use the Repository Pattern in Flutter
The Repository pattern serves as a critical architectural component in Flutter development, especially when dealing with complex data management and ensuring the scalability of an application.
Its implementation is guided by the unique requirements of each project, with particular emphasis on the abstraction of data sources and the simplification of data manipulation across the application.
Understanding the Appropriate Context for the Repository Pattern
The Repository pattern is most beneficial in scenarios where an application interacts with multiple data sources. It abstracts the complexities associated with these varied data sources, allowing for a more streamlined and manageable codebase. This pattern proves invaluable in projects that require a high degree of modularity and flexibility in data handling, such as those involving offline data access, synchronization, and caching mechanisms. Its adoption is particularly recommended when the goal is to enhance the testability of the application, facilitating the mocking of data sources and enabling more efficient unit testing practices.
How to Implement The Repository Pattern
The implementation process begins with the definition of repository interfaces. These interfaces are crucial for delineating the contract between the data layer and the rest of the application, ensuring that changes to the implementation details of data access mechanisms do not impact the application’s business logic.
Following the establishment of these interfaces, the next step involves the creation of concrete classes that implement these interfaces. These concrete repository classes are responsible for the actual data operations, such as fetching data from APIs, reading from or writing to local databases, and managing caching logic.
To maintain a decoupled architecture, it’s advisable to employ dependency injection techniques. Dependency injection allows for the dynamic provision of repository instances to the components that require them, significantly enhancing the flexibility and modularity of the application.
This approach not only simplifies the process of swapping out implementations for testing purposes but also contributes to a cleaner and more organized code structure.
The final step in leveraging the Repository pattern within a Flutter application involves the integration of these repositories into the application’s business logic. This integration ensures that the application’s core functionality interacts with data through a uniform and simplified interface, provided by the repositories.
This methodological approach to data management facilitates the development of applications that are robust, maintainable, and capable of adapting to changing data sources or business requirements with minimal impact on the overarching application architecture.
Abstract vs. Concrete Classes in Repositories
The choice between abstract and concrete classes in the context of repositories in software development, including Flutter applications, is a subject of considerable debate. This decision can significantly impact the design, flexibility, and testability of the application. Understanding the advantages and use cases for each approach can help developers make informed decisions tailored to their specific project needs.
Abstract Classes in Repositories
Advantages:
- Abstract classes provide a high level of flexibility by defining a set of methods that the concrete classes must implement. This flexibility allows developers to change the underlying data source or logic without affecting the rest of the application.
- Testing is more straightforward with abstract classes because they enable easy mocking or stubbing of repository implementations. This is particularly beneficial when unit testing business logic that depends on data managed by repositories.
- By defining a clear contract for repositories, abstract classes ensure consistency in how data is accessed and manipulated across different parts of the application.
Use Cases:
- For applications that interact with multiple data sources, abstract classes allow for the easy interchange of implementations without modifying the dependent code.
- In scenarios where the data access layer may need to evolve independently from the rest of the application, abstract repositories provide a stable interface while allowing the underlying implementation to change.
Concrete Classes in Repositories
Advantages:
- Concrete classes can be simpler to implement and understand, especially in smaller projects or when the data access logic is straightforward. They eliminate the need for an additional layer of abstraction, making the codebase more concise.
- In some cases, using concrete classes can lead to slightly better performance, as there’s one less abstraction layer. However, this advantage is usually negligible in the context of most applications.
- Concrete classes allow developers to write specific implementations directly, which can be advantageous when the application requires a very tailored approach to data handling that does not fit well with a generalized abstract class.
Use Cases:
- When an application has a straightforward data model or does not require the flexibility of switching between different data sources, concrete classes offer a direct and uncomplicated approach.
- During the initial phases of development, when the focus is on quickly validating ideas, concrete classes can accelerate development by reducing the overhead of managing abstract classes and their implementations.
Balancing the Decision
The choice between abstract and concrete classes in repositories often comes down to a balance between the need for flexibility, testability, and consistency (favoring abstract classes) versus the desire for simplicity and directness (favoring concrete classes). For large-scale, complex applications with multiple data sources and a need for high testability, abstract classes are generally preferred. Conversely, for smaller projects or when rapid development and simplicity are priorities, concrete classes may be more appropriate.
Strategies for Testing Repository Implementations
1. Isolate the Repository Layer: The first step in testing repositories is to isolate them from the rest of the application. This isolation allows for targeted tests that focus specifically on the functionality of the repositories without interference from other app components. Isolation is achieved by mocking or stubbing the dependencies of the repository, such as data sources or other services.
2. Define Test Cases: Define a comprehensive set of test cases that cover all the expected behaviors of the repository. This includes successful data retrieval and storage, handling of errors or exceptions, and edge cases. The goal is to ensure that the repository correctly interacts with its data sources and adheres to the defined business rules.
3. Use a Testing Framework: Leverage a testing framework that supports the mocking and assertion capabilities needed to effectively test repositories. For Flutter, popular choices include the flutter_test package for unit and widget tests, along with mockito or mocktail for mocking dependencies.
Also, read Implementing clean architecture with Getx for building scalable and maintaianable applications.
Mocking Data Sources for Unit Tests
1. Mocking Dependencies: Use a mocking library like mockito or mocktail to create mock versions of the data sources the repository interacts with. Mock objects can simulate various scenarios, such as data retrieval, failure responses, or network errors, without making actual calls to a database or network service.
2. Simulating Data Responses: Configure the mock data sources to return specific responses when their methods are called. This allows you to test how the repository handles different types of data and situations, such as empty data sets, valid data, or error conditions.
3. Testing Success Scenarios: Write unit tests that verify the repository’s behavior when data retrieval or storage operations succeed. Assert that the repository returns the expected data or performs the expected actions, such as caching data locally.
4. Testing Error Handling: Equally important are tests that simulate error conditions, such as network failures or corrupt data. These tests should ensure that the repository gracefully handles errors, either by retrying operations, returning fallback values, or propagating errors to be handled by the calling code.
5. Verifying Interactions with Data Sources: Beyond testing the outcomes of repository methods, it’s also valuable to verify that the repository interacts with its dependencies as expected. Use the mocking library’s capabilities to assert that certain methods were called with the correct parameters, ensuring that the repository correctly delegates tasks to its data sources.
Advanced Repository Patterns and Practices
Implementing repositories in your Flutter applications not only streamlines data management but also introduces complexity, especially when it comes to error handling, scalability, and simplicity. Let’s delve into advanced repository patterns and practices that address these aspects, ensuring your repositories are robust, scalable, and maintainable.
Handling Errors and Exceptions in Repositories
Robust error handling within repositories is crucial for building resilient applications. Repositories act as the intermediary between your application’s core logic and the data layer, making them a critical point for managing errors and exceptions that arise during data operations.
Best Practices for Robust Error Handling:
- Explicit Error Types: Define clear and explicit error types for different kinds of failures that can occur in your repositories. This approach aids in handling errors more effectively at higher levels of your application, such as showing specific error messages to the user.
- Try-Catch Blocks: Use try-catch blocks judiciously within your repository implementations to catch exceptions at the point of failure. This allows for logging errors, performing cleanup, and converting exceptions into meaningful error types.
- Error Propagation: Propagate errors up to the calling code, allowing it to decide on the best course of action (e.g., retrying the operation, showing an error message). Avoid swallowing errors silently within your repositories.
- Consistent Error Handling Strategy: Maintain a consistent strategy for handling errors across all repositories. This consistency helps in reducing the cognitive load on developers and makes the codebase easier to maintain.
Repositories Scale Horizontally
As your Flutter application grows, so does the complexity and volume of data it handles. Scaling repositories effectively becomes essential to accommodate this growth without degrading performance or maintainability.
Techniques for Scaling Repositories as Your App Grows:
- Modularization: Break down large repositories into smaller, more focused repositories. This modular approach makes it easier to manage and scale the data layer as your application evolves.
- Caching: Implement caching strategies within your repositories to reduce the load on your primary data sources and improve the responsiveness of your application.
- Asynchronous Operations: Leverage asynchronous operations and concurrency management to handle large volumes of data or long-running data operations without blocking the user interface.
Keeping Your Repository Implementation Simple
While repositories are powerful, there’s a risk of over-engineering them with too many features or complex data handling logic. Keeping your repository implementation simple and focused is key to maintaining a clean and maintainable codebase.
Tips for Avoiding Over-Engineering and Maintaining Simplicity:
- Focus on Abstraction: Ensure your repositories provide a clear and minimal interface for the data operations needed by the rest of your application. Avoid adding unnecessary functionality that isn’t directly required.
- Leverage Flutter and Dart Features: Use the features and utilities provided by Flutter and Dart, such as streams and futures for asynchronous data handling, to simplify your repository implementations.
- Refactor as You Go: Regularly refactor your repository implementations as your application evolves. This continuous refinement helps in identifying and removing unnecessary complexities or duplications.
Maintaining and Scaling Flutter Clean Architecture
Maintaining and scaling Flutter Clean Architecture as projects evolve is crucial for ensuring that applications remain efficient, manageable, and adaptable to new requirements or changes.
As applications grow in complexity and size, adhering to Clean Architecture principles becomes both a challenge and a necessity. Here are strategies, tools, and practices designed to help developers maintain and effectively scale their Flutter applications while keeping the architecture clean and organized.
Maintaining Clean Architecture
1. Continuous Refactoring
Regularly review and refactor your code to align with Clean Architecture principles. This includes simplifying complex classes, breaking down large modules into smaller, more manageable pieces, and ensuring that dependencies are correctly managed and injected.
2. Adherence to SOLID Principles
The SOLID principles are foundational to Clean Architecture. By ensuring that your Flutter application adheres to these principles, you can maintain a high level of modularity, flexibility, and reusability in your codebase.
3. Automated Testing
Implement a comprehensive suite of automated tests, including unit, integration, and end-to-end tests. Automated testing is essential for maintaining the integrity of your application’s architecture as it evolves, allowing for confident refactoring and additions.
4. Modularization
Organize your Flutter project into modules or packages that encapsulate specific functionalities or features. Modularization aids in maintaining a clean separation of concerns and makes it easier to manage dependencies and scale the application.
Scaling Flutter Clean Architecture
1. Dynamic Feature Modules
For larger applications, consider using dynamic feature modules that can be loaded on demand. This approach helps in scaling the application by reducing the initial load time and minimizing the memory footprint.
2. Effective State Management
Choosing the right state management solution is crucial for scaling. Options like Provider, Riverpod, Bloc, or MobX can help manage application state in a scalable and maintainable way, ensuring that your architecture remains clean and efficient.
3. Dependency Injection (DI)
Utilize DI frameworks, such as GetIt or Riverpod, to manage dependencies in your Flutter application. DI aids in decoupling components, making it easier to scale and maintain the application while adhering to Clean Architecture principles.
4. Performance Optimization
As your application scales, maintaining and optimizing performance becomes increasingly important. Utilize Flutter’s performance profiling tools to identify and address bottlenecks. Implement lazy loading, caching, and other optimization techniques to ensure that your application remains responsive and efficient.
Also, read Implementing clean architecture with Getx for building scalable and maintaianable applications.
5. Continuous Integration/Continuous Deployment (CI/CD)
Implement CI/CD pipelines to automate testing, building, and deployment processes. CI/CD helps in maintaining code quality and ensures that new features or changes do not introduce regressions, facilitating smoother scaling of your application.
6. Architectural Reviews
Conduct regular architectural reviews with your development team to assess the alignment of the application with Clean Architecture principles. Use these reviews as an opportunity to identify areas for improvement, simplification, or refactoring.
Conclusion
Flutter Clean Architecture and effectively implementing repositories isn’t just about following a set of rules; it’s about creating a foundation for your apps that ensures they’re robust, maintainable, and scalable.
Whether you’re abstracting data sources with repositories, refactoring for better modularity, or scaling up with advanced patterns, the journey towards a cleaner architecture is ongoing.
The goal is not perfection on the first try but continuous improvement. Keep testing, keep refining, and don’t shy away from revisiting your architecture as your app evolves. Embrace the principles of Clean Architecture, and you’ll find that managing and scaling your Flutter projects becomes a more streamlined and, dare I say, enjoyable process. Happy coding!
FAQ’s
What is the role of a repository in Clean Architecture?
In Clean Architecture, the repository plays a crucial role as a mediator between the data layer and the domain layer. It abstracts the origin of the data that the application uses, allowing for a clean separation of concerns. By doing this, repositories enable the application’s core logic to remain independent of data sources, such as databases or web services. This separation enhances maintainability, scalability, and testability, as business logic can evolve independently of the underlying data sources.
What are the benefits of repository architecture?
- Decoupling: It separates the data access logic from the business logic, reducing dependencies.
- Abstraction: It abstracts the data source from the application, making it easier to switch data sources without affecting the business logic.
- Maintainability: Simplifies the maintenance of the codebase by isolating the data layer.
- Testability: Facilitates unit testing of the business logic by mocking the data access layer.
- Scalability: Supports the growth of the application by allowing efficient data management strategies.
Why use Clean Architecture in Flutter?
Using Clean Architecture in Flutter applications ensures a scalable, testable, and maintainable codebase. It facilitates the separation of concerns by dividing the application into layers with defined responsibilities. This structure enhances the development process, making it easier to manage complex features, improve code quality, and facilitate team collaboration. Additionally, it allows for the flexible integration of new technologies and data sources, making your Flutter apps more adaptable to change.
What is the purpose of the repository layer?
The purpose of the repository layer in software architecture is to provide a clean abstraction over the data layer, enabling the application to access and manipulate data without being concerned about the specifics of the data storage mechanisms. This layer acts as a bridge between the domain and data mapping layers, helping to keep the domain model and business logic separate from the data model. This separation of concerns ensures that changes to the database or the nature of the data source have minimal impact on the domain logic, improving the application’s maintainability and flexibility.
Why do I need a repository?
- Abstraction: It abstracts the complexity of data operations, providing a simpler interface for data access.
- Separation of Concerns: It separates the data access logic from the business logic, making the code cleaner and more manageable.
- Flexibility: It makes the application more flexible, allowing changes to the data storage without impacting the business logic.
- Testability: It enhances the testability of the application by allowing business logic to be tested independently of data access mechanisms.
- Maintainability: It improves code maintainability, making future changes and debugging easier.