In Flutter development, where clean architecture helps us build apps that are easy to maintain and scale. But, no matter how well we plan, errors can still pop up and cause problems.
In this guide, we’re going to learn how to handle those errors effectively in Flutter’s clean architecture. This will make sure our apps work smoothly and can deal with issues without any hassle.
Whether you’re new to Flutter or have been using it for a while, understanding error handling in flutter clean architecture is key to making great apps. Let’s dive in and make our Flutter projects even better!
A short Overview of Clean Architecture in Flutter
Clean architecture is all about organizing your Flutter app in a way that separates concerns, making it easier to manage and test. Think of it as dividing your project into layers, each with its own job.
- Presentation Layer: This is where your UI lives. It’s what your users see and interact with. Here, you display data to the user and handle their inputs.
- Domain Layer: Consider this the brain of your app. It contains the business logic, rules, and models. This layer decides how your app should work, independent of the UI.
- Data Layer: This is where your app interacts with external sources like databases and web services. It fetches, stores, and manages external data.
Using clean architecture helps keep your code organized, making it easier to update and debug. Plus, it prepares your app to handle changes and growth smoothly.
Now, why is error handling important here? Well, by implementing clean architecture in Flutter with effective error handling strategies across each layer, we ensure that our app can gracefully manage and recover from unexpected situations, enhancing the user experience and app reliability.
Let’s see how we can apply these principles to make our Flutter apps more robust.
Common Errors in Flutter Applications
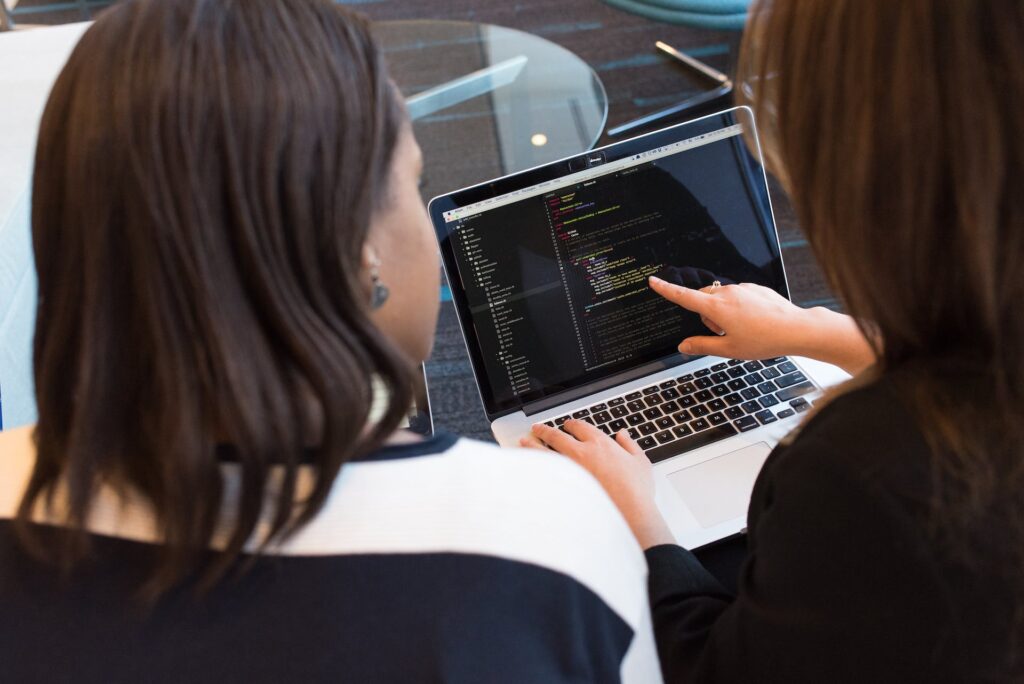
In Flutter applications, errors can usually be categorized into three main types, each affecting the optimization of app’s performance and user experience in different ways. Understanding these errors helps in creating a solid error handling strategy.
Here’s the common flutter application error:
- Compilation errors
- Runtime errors
- Logical errors
Compilation Errors
These happen when there’s an issue in the code that prevents the app from being compiled. It could be due to syntax mistakes, type mismatches, or missing resources. Compilation errors need to be fixed in the development phase before the app can run.
Runtime Errors
These occur while the app is running. They can be caused by various issues like null pointer exceptions, accessing a list out of its bounds, or failing to load a necessary resource. Runtime errors can crash the app if not handled properly.
Logical Errors
These are the trickiest to spot because the app doesn’t crash or show any obvious signs of failure. Logical errors happen when the code doesn’t perform as expected, leading to incorrect outcomes. They can be due to flawed logic or incorrect assumptions.
If you want to learn flutter app development we created a free Flutter firebase bootcamp, In this course you will learn step by step process to make an application using clean architecture from scratch.
Principles of Effective Error Handling
Effective error handling in Flutter’s clean architecture is not just about catching errors; it’s about designing your app to manage and recover from errors gracefully.
Here are some key principles to guide you
Prevention is Better than Cure
The first line of defense against errors is to prevent them from happening. This involves writing clean, simple, and tested code. Use static analysis tools to catch potential errors early in the development process.
Catch and Manage Errors Gracefully
When errors do occur, ensure your app can catch them without crashing. Use try-catch blocks strategically to handle potential errors and provide fallbacks. For example, if a network request fails, you might show a cached version of the data or a friendly error message.
Provide Meaningful Feedback to Users
Instead of showing generic error messages like “Something went wrong,” provide users with clear and actionable information. If the error is recoverable, guide them on what to do next. This improves the user experience even when things don’t go as planned.
Log Errors for Analysis
Logging errors is crucial for understanding and fixing them. Use logging tools to record errors as they happen. This data is invaluable for diagnosing issues that users encounter in the wild.
Regularly Review and Refine Error Handling Strategies
As your app evolves, so should your error handling strategies. Regularly review your app’s error logs and user feedback to identify areas for improvement.
Also, read Implementing clean architecture with Getx for building scalable and maintaianable applications, and clean architecture with TDD.
Implementing Error Handling in Flutter Clean Architecture
Implementing effective error handling in Flutter’s clean architecture involves addressing errors at each layer of the architecture—Presentation, Domain, and Data. Here’s how to approach error handling in each layer:
Error Handling in the Presentation Layer
Use Try-Catch Blocks
Surround code that can potentially throw errors with try-catch blocks. This is particularly important for operations like fetching data from the internet or reading files from the device’s storage.
Display User-Friendly Error Messages
When an error occurs, catch it and display a meaningful message to the user. Avoid technical jargon. Instead, explain what happened and suggest a next step if possible. For instance, if a network request fails, you might say, “Unable to connect. Please check your internet connection and try again.”
Error Handling in the Domain Layer
Define Custom Exceptions
Create custom exception classes for errors that can occur in your business logic. This makes it easier to handle specific errors in a more meaningful way.
Use Error Handling in Use Cases
When writing use cases, anticipate possible errors and handle them appropriately. This might involve returning either a success result or an error result, which the Presentation layer can then act upon.
Error Handling in the Data Layer
Handle API Errors
When making API requests, properly handle responses that indicate an error, such as a 404 or 500 status code. Convert these into exceptions or error objects that the rest of your app can understand and react too.
Manage Database Errors
When interacting with a database, catch errors that could occur during operations like reading, writing, or updating data. Handle these errors gracefully by, for example, retrying the operation or providing a fallback solution.
Strategies for Effective Error Handling Across Layers
Centralized Error Handling
Consider implementing a centralized error handling mechanism that can catch and manage errors across all layers. This can help reduce code duplication and make your error handling logic more consistent.
Asynchronous Error Handling
For operations that are asynchronous, such as network requests or database operations, use Futures and async-await syntax in Dart to handle errors. Make sure to use try-catch blocks around asynchronous calls to catch exceptions.
User Feedback and Recovery Options
Whenever possible, provide users with options to recover from an error. This could be retrying a failed operation, resetting the application state, or contacting support for help.
Error Logging and Monitoring
In a well-structured Flutter application following clean architecture, error logging and monitoring play a crucial role in maintaining app health and enhancing user experience. Here’s how you can implement these practices effectively:
Importance of Logging Errors
- Understanding the Context: Error logs provide valuable insights into the circumstances under which an error occurred, helping developers diagnose and fix issues more efficiently.
- Proactive Problem Solving: Regularly reviewing error logs allows teams to identify and address potential issues before they affect a significant number of users.
Tools and Practices for Monitoring Errors in Flutter Apps
- Use Logging Packages: Flutter offers several packages for logging, such as logger and flutter_logs, which can be integrated into your app to capture detailed logs. These tools allow for customizable log levels (e.g., debug, info, warning, error) and can format output for easier analysis.
- Remote Monitoring Services: Consider integrating with remote monitoring services like Firebase Crashlytics, Sentry, or LogRocket. These services can capture real-time error data, aggregate logs, and alert you to issues as they happen, providing dashboards for tracking error frequencies, affected user segments, and more.
- Local vs. Remote Logging: While local logging is useful during development and debugging, remote logging is essential for capturing errors that occur in production. Ensure your logging strategy covers both scenarios.
Analyzing Error Logs to Improve App Stability
- Identify Patterns: Look for patterns or commonalities among errors, such as specific devices, OS versions, or app versions that are more prone to certain types of errors.
- Prioritize Based on Impact: Use the data from your logs to prioritize fixes based on the impact on users and the frequency of errors.
- Continuous Improvement: Make error log analysis a regular part of your development cycle. Use insights gained from logs to inform testing strategies and prevent similar issues from occurring in the future.
Best Practices for Error Logging and Monitoring
- Privacy and Security: Ensure that your logging practices comply with data protection laws and do not inadvertently log sensitive user information.
- Efficient Data Management: Set up log rotation and archiving policies to manage log data efficiently, ensuring that older logs are archived or deleted to free up storage while keeping important data accessible for analysis.
- User Feedback Integration: Encourage users to report errors or issues they encounter, providing an easy way to include screenshots or logs. This user-reported data can be invaluable for identifying issues not caught by automated logging.
For Security best practices must read, Security best practices in Flutter clean architecture.
Best Practices for Error Handling in Flutter Clean Architecture
To ensure your Flutter app not only handles errors gracefully but also maintains a clean architecture, here are some best practices to follow:
Centralized Error Handling Logic
- Implement a central mechanism for handling errors to avoid duplicating error handling logic across the app. This can be achieved by using error handling mixins or services that can be called from anywhere in the app.
- Define a standard way of propagating errors from the Data layer up to the Presentation layer, ensuring consistency in how errors are managed and displayed.
Custom Error Classes and Handling Frameworks
- Create custom error classes that encapsulate specific error states relevant to your app’s domain. This approach offers more granularity and control over error handling compared to using generic exceptions.
- Consider using or developing a framework that standardizes how errors are caught, logged, and handled across different layers of your app. This can simplify error management and make the codebase more maintainable.
Testing Strategies for Error Handling
- Write unit and integration tests that specifically test error handling paths in your code. Ensure your tests cover scenarios like network failures, invalid input, and unexpected data formats.
- Utilize Flutter’s testing framework to simulate errors and exceptions, verifying that your app behaves as expected under error conditions.
User Experience Considerations
- Always keep the user informed about what’s happening, especially during errors. Use loading indicators, progress bars, and informative error messages to enhance the user experience.
- Provide clear recovery paths for users when errors occur. This could be in the form of retry buttons, contacting support, or steps to correct the input they have provided.
Continuous Monitoring and Improvement
- Use analytics and monitoring tools to track error rates and user impact. Analyze this data regularly to identify trends and prioritize fixes.
- Adopt a continuous improvement mindset. As your app evolves, so should your error handling strategies. Use feedback from users and crash reports to refine and update your approach.
Implementing These Practices
Implementing these best practices requires a thoughtful approach that balances technical robustness with user experience. Start by reviewing your current error handling mechanisms and identify areas for improvement.
Gradually integrate these practices into your development workflow, prioritizing changes that have the most significant impact on app stability and user satisfaction.
Tools and Libraries to Aid Error Handling in Flutter
When building Flutter applications with clean architecture or MVVM, leveraging the right tools, repositoriesv and libraries can significantly streamline error handling processes. Here’s a rundown of some popular options that can aid in error handling in clean architecture in flutter, along with a comparison of their features:
Popular Flutter Packages for Error Handling
- Dio: Dio is a powerful HTTP client for Dart/Flutter, which supports Interceptors, Global configuration, FormData, Request Cancellation, File downloading, Timeout, etc. It’s particularly useful for managing network errors and customizing error handling for HTTP requests.
- Flutter Bloc: While primarily a state management solution, Flutter Bloc can be used to handle errors gracefully in the presentation layer. It allows developers to respond to state changes, including error states, in a predictable manner.
- Sentry: Sentry provides real-time error tracking that gives you insight into production deployments and information to reproduce and fix crashes. It’s great for capturing exceptions and errors in your Flutter apps and diagnosing them with stack traces and context.
- Firebase Crashlytics: A powerful real-time crash reporter that helps you track, prioritize, and fix stability issues that erode your app quality. Firebase Crashlytics saves you troubleshooting time by intelligently grouping crashes and highlighting the circumstances that lead up to them.
Comparing Their Features
Feature/Tool | Dio | Flutter Bloc | Sentry | Firebase Crashlytics |
Network Errors | Yes | No | Yes | Yes |
Error Tracking | No | No | Yes | Yes |
Real-Time Reporting | No | No | Yes | Yes |
State Management | No | Yes | No | No |
Custom Error Handling | Yes | Yes | Yes | Yes |
How to Integrate Them into Your Project
- Dio for Network Errors: Wrap your network requests in Dio and use its interceptors to catch and handle errors. You can easily map Dio exceptions to your custom error classes.
- Flutter Bloc for State Management: Use Bloc to manage error states in your UI. Define error states and emit them when errors occur, allowing your UI to respond accordingly.
- Sentry and Firebase Crashlytics for Error Tracking: Integrate Sentry or Firebase Crashlytics into your Flutter project to capture and analyze errors. Both tools offer SDKs that are easy to set up and start sending error data.
Conclusion
Handling errors in Flutter clean architecture smart can make the apps more reliable and user-friendly. We’ve covered how to organize your app with clean architecture and tackle errors at each layer. The goal is to keep your app running smoothly and keep your users happy, even when things go wrong.
Use the tools and tips we talked about to catch and fix errors, and always think about how to make things clear for your users. Error handling is a big part of making great apps, so keep learning and improving. Thanks for sticking with us through this guide to making your Flutter apps better!
FAQ’s
What is error handling in clean code?
Error handling in clean code refers to the practice of writing code that anticipates, detects, and responds to errors or exceptions in a way that maintains the readability and maintainability of the codebase. It involves using structured approaches to deal with runtime errors, ensuring the program can handle unexpected inputs or situations gracefully without crashing or producing incorrect results.
What is the error handling widget in Flutter?
In Flutter, the ErrorWidget is a widget that displays an error message. It’s used when the framework fails to build a widget, allowing developers to provide a user-friendly error message. Developers can customize Flutter’s error handling behavior by setting the ErrorWidget.builder to display custom error messages or designs.
What is the error handling process?
The error handling process involves identifying errors, capturing them, and responding to them appropriately. This process includes anticipating potential errors, detecting when they occur, handling them gracefully (e.g., through retries, logging, user notifications), and implementing corrective measures to prevent future occurrences. It aims to ensure the application remains robust and user-friendly despite unexpected conditions.
How do you handle error response in Flutter?
To handle error responses in Flutter, you can use try-catch blocks to catch exceptions when making network requests or accessing resources that might fail. Additionally, you can use FutureBuilder or StreamBuilder widgets to manage asynchronous operations and specify custom widgets to display in case of errors, ensuring a smooth user experience even when errors occur.
What are the three error handling techniques?
The three primary error handling techniques are:
- Try-Catch-Finally: Used to catch exceptions in a controlled block of code, with an optional finally block for cleanup.
- Throwing Exceptions: Creating and throwing custom exceptions to signal error conditions to calling functions.
- Error Callbacks: Using callback functions that are invoked in case of errors, commonly used in asynchronous programming.
Why do we need error handling code?
Error handling code is essential to ensure the reliability and robustness of software. It allows programs to deal with unexpected conditions or inputs gracefully, preventing crashes and protecting the system from potential security vulnerabilities. Proper error handling improves the user experience by providing meaningful feedback and ensures that the application can recover from errors without losing data or functionality.